Welcome, intrepid explorers of the code abyss, fellow cosmic travelers who dare to delve into the whimsical, wondrous world of TypeScript interface generics! Indeed, you stand upon the threshold of enlightenment regarding the delightful dance of parameterized types and their exquisite synergy with reusable interfaces. Gather โround, as we embark on an odyssey of thoughtโprepare for your neurons to tingle and your creativity to soar! Today, weโll unlock the secrets, the hidden services of TypeScript interface generics, an arcane yet vital tool in the arsenal of modern developers!
Reusability, my fellow code alchemists, is the quintessence of programming efficiency! An interface spills forth its bountiful possibilities, but alas, static it remains if we lack the genericsโthose shimmering parameters that allow us to inject type magic into our structures. We are, after all, no mere mortals clutching a single type like a crutch; we must adopt the heroic mantle of polymorphism! By embracing TypeScript interface generics, we become the fearless architects of adaptabilityโour interfaces transcending the mundane, evolving to suit the ever-shifting needs of developersโ burgeoning creations.
As we venture nearer into this synthesized concoction of types and interfaces, one must not forget the subtleties of implementation. For it is here, dear coders, that the true artistry lies! One may create a generic interface in TypeScript with astounding ease. Lo and behold, the syntax itself! An elegant expression like a sonnet, you might declare:
```typescript
interface Box {
contents: T;
}
```
Ah, but the beauty of this concise declaration! โT,โ the magical variable leaping from the confines of bound types! Allowing a Box to contain integers, strings, or even our beloved objectsโitโs an awe-inspiring exhibition of versatility! And just when you thought you grasped its simplicity, gasping in delight, consider how it extends to beyond mere storage, crafting a conduit through which our data flows freely!
Alas, my fellow travelers, the stage is set, the curtain poised to rise! As we unfurl this exploration further, where ensure you remain with bated breathโa panorama of various use cases awaits! From repositories that cater to different objects gracefully dancing in harmony, to services that summon forth the magic of generics for dynamic data manipulation.
We shall uncover the alchemy contained within combining classes with interface generics, revealing a fusion that promises new levels of functionality and elegance in your code. Careful, brave adventurersโmistress TypeScript has secrets known only to those bold enough to wield her power!
To wrap this layer of complexity, let us not overlook the implications of employing TypeScriptโs generics in real-world applications. How they serve as the glue binding together the parts, creating a seamless operation within our ever-evolving software landscapes.
Unleashing the Power of TypeScript Interface Generics
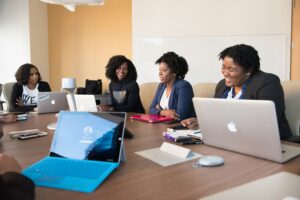
Behold the majestic realm of TypeScript interface generics, a kaleidoscope of possibilities flickering and swirling like an over-caffeinated butterfly in a computer science lab! Imagine, if you will, the manipulation of types, the glorious dance of parameters and polymorphism intertwined within the tapestry of your code. The sheer brilliance of type abstraction awaits, eager to elevate reusability to dizzying heights within the boundaries of your creative coding exploits.
The Art and Science of Parameterization
Ah, parameters โ the enchanting breadcrumbs of our computational journey! With TypeScript interface generics, we wield the ability to craft templates that morph and shift, adapting to our whims and desires. What an exhilarating experience it is to declare an interface as general, like a chameleon blending seamlessly into the lush foliage of our programming projects!
```typescript
interface Box {
contents: T;
getContents(): T;
}
```
Now, picture this: an interface named `Box`, a simple enough vessel, isn’t it? But lo and behold! Thanks to the whimsical nature of TypeScript interface generics, you can create boxes for every type of content imaginable! Numbers, strings, objects, or even those peculiarly strange ones that we call functions โ the boundaries of your code expand like an ever-growing universe, allowing you to build versatile services.
Consider, dear reader, how you might harness the power of this interface in the midst of your polymorphic symphony! With its newfound flexibility, you could write a function as charming as a cat scurrying across your keyboard, eager to take any `Box` as its playful subject and dance with it in a whirl of type safety.
```typescript
function unwrap(box: Box): T {
return box.getContents(); // Ah, the thrill of polymorphic potential!
}
```
A function named `unwrap` making its grand entrance, how delightful! It elegantly extracts contents from any box, regardless of its inner type. Isnโt it marvelous how our `unwrap` function employs the generics to embrace every whim of the code? The beauty of reusability, my fine friends, emerges like a phoenix from the ashes of inflexibility!
Embrace the Generics Paradigm with Functions
Dare we delve deeper into the murky waters of TypeScript interface generics? Indeed! Ours is a quest fueled by curiosity and caffeine! Let us embark on a journey through functional territories where we can consider generics not merely as a means to an end but as magical stepping stones towards a more robust and maintainable codebase.
Imagine a scenario where you rule over a kingdom of services โ yes, services, the most regal of architectural patterns! You have a collection of services, each demanding a litany of parameters but sharing a common characteristic: they all need to accept and return various types of data. Here enters the hero of our narrative, the TypeScript interface generics, to rescue your weary soul from tedious repetitiveness!
```typescript
interface ApiService<T, R> {
request(data: T): R;
}
```
What do you see? A gleeful proclamation! An interface named `ApiService`, orchestrating requests with two generic types! The `T` and `R` stand tall, representing the input type and the return type, respectively. Ah, the grandeur of abstraction! Services crafted upon this interface can seamlessly accept sponsorship from a myriad of data types, deftly defying the limitations of convention.
Let’s sample the divine chocolate decadence of our creations. A maximalist content request service might revel in the richness of objects, while a minimalist response service could sing the praises of primitive types!
```typescript
class UserService implements ApiService<{ username: string }, { success: boolean }> {
request(data: { username: string }): { success: boolean } {
// Hereโs a hasty mock-up of an actual API request!
return { success: true }; // Now, bow down to the wizardry of generics!
}
}
```
Kudos to the `UserService` for diminishing redundancy! By embracing TypeScript interface generics, it can eagerly accept any parameterized request, transforming form into function without ever losing sight of its mission. Code reusability has been redefined within this sparkling realm; the boundaries swelling like the tides, while consistency remains triumphant.
The Synthesized Euphoria of Reusability
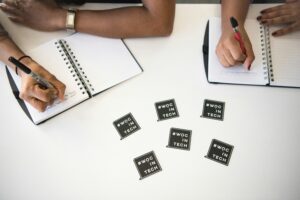
Now, let’s gather the fragments of our wondrous journey! Returns, requests, boxes, and the beautiful transformation of plain interfaces into polymorphic palaces! This glorious playground of TypeScript interface generics imbues our projects with the exhilarating fragrance of reusability!
When designed thoughtfully, you can deploy an archetypal structure where each function dances to the rhythm of its parameters, effortlessly adapting and molding to suit the ever-changing
Wrapping Up the Exploration of TypeScript Interface Generics
In the vast ocean of programming paradigms, where the winds of change continuously reshape the shores of software development, TypeScript interface generics stand as a lighthouse, guiding developers through an intricate labyrinth of types, contracts, and components. They emerge not merely as a tool for abstraction but as a potent instrument for fostering flexibility within the codebase. By providing a means to define templates that adapt to various data types while imposing essential constraints and bounds, TypeScript interface generics allow us to elevate our coding practices to levels hitherto unimagined.
The Potent Synergy of Flexibility and Constraints
To understand the profound impact of TypeScript interface generics, one must appreciate the delicate balance they strike between flexibility and constraints. Imagine, if you will, a chef in a kitchen that, while equipped with an array of ingredients (the types), must adhere to specific dietary requirements (the constraints). The generics act as a recipe that adapts effortlessly to these unique conditions, crafting dishes (components) that accommodate everything from vegetarian to gluten-free preferences. This seamless adaptability in TypeScript enables developers to harness the full power of abstraction, ensuring that our components remain robust, reusable, and maintainableโa tantalizing vision of code perfection.
When we delve deeper into the theme of constraints, the narrative grows richerโthe notion that while the expansiveness of generics gives us the freedom to work with diverse types, these types must also adhere to certain predefined contracts. These contracts, rather than being shackles that bind creativity, serve as the scaffolding upon which our generics can flourish. Think of them as the parameters of an art project: they provide guidance and direction, allowing the artist (the developer) to express creativity within a defined framework. This symphony of structure and freedom is where TypeScript interface generics shine, illuminating the path toward constructing reliable and versatile services.
Elevating Component Design and Enhancement
As we traverse the landscape of modern application development, adaptability and evolution emerge as non-negotiable prerequisites for any successful project. Herein lies the beauty of TypeScript interface generics: the power to evolving component design. Just as a finely-tuned machine can pivot to meet the demands of a changing marketplace, generics empower our components with the ability to morph and adapt without breaking the integrity of the underlying system.
Consider the world of web applications, where components must often pull data from a variety of sources, each with its own unique structure and type. By leveraging TypeScript interface generics, we create services that are not only resilient but also elegantly malleable. A component designed with generics can seamlessly integrate with a multitude of data formats, which means that developers are no longer confined to strict type definitions. Instead, they can enthusiastically embrace the fluidity of data interchange, ensuring that our applications remain relevant and functional amidst the ever-shifting sands of user needs and technological advancements.
But there is moreโwithin the dance of constraints and adaptable structures, another significant advantage materializes: enhanced collaboration. In a team of developers, where communication can sometimes resemble chaos, the introduction of generics becomes a unifying force. Every team member collaborates under a shared understanding of the contracts defined by the generics, thus minimizing ambiguity and fostering a culture of clarity. The risk of misinterpretation thus diminishes as each developer interacts with components that possess a clear and defined purposeโa well-orchestrated performance that is both coherent and effective.
As we land on this broad canvas, intertwined with the threads of theory and practice, it becomes increasingly clear that TypeScript interface generics are more than mere constructs; they are the very bedrock upon which modern service-oriented architecture rests. They breathe life into our code, infusing it with a sense of purpose and direction. When we engage with generics, we are not just writing lines of code; we are weaving intricate patterns that will shape the future of our applications.
Concluding Thoughts
In conclusion, the exploration of TypeScript interface generics unveils a heroic tale of flexibility, constraints, and collaborative success. We, the architects of code, wield this tool with the understanding that our creations will evolve, adapt, and flourish in a digital ecosystem that is in constant flux. As we embrace the ability of generics to define clear contracts and create resilient components, we also embrace a future where our services become seamless extensions of the users’ needsโa narrative that not only strengthens our coding practices but also enhances the overall user experience.
As you embark on your journey with TypeScript interface generics, may you remain ever-curious, ever-ambitious, and ever-ready to craft exceptional services that adhere to the principles of flexibility and constraint, ensuring that every line of code you write echoes with the promise of possibility.